网站首页 > 技术文章 正文
要在Python中读写SQL Server数据库,您可以使用pyodbc库。
以下是一个示例代码,演示如何连接到SQL Server数据库并执行读写操作:
import pyodbc
# 连接到SQL Server数据库
def connect_to_sql_server():
conn = pyodbc.connect(
'Driver={SQL Server};'
'Server=your_server_name;'
'Database=your_database;'
'UID=your_username;'
'PWD=your_password;'
)
return conn
# 执行查询语句
def execute_query(conn, query):
cursor = conn.cursor()
cursor.execute(query)
result = cursor.fetchall()
cursor.close()
return result
# 执行插入语句
def execute_insert(conn, query, values):
cursor = conn.cursor()
cursor.execute(query, values)
conn.commit()
cursor.close()
# 示例用法
conn = connect_to_sql_server()
# 执行查询
select_query = "SELECT * FROM your_table"
result = execute_query(conn, select_query)
for row in result:
print(row)
# 执行插入
insert_query = "INSERT INTO your_table (column1, column2) VALUES (?, ?)"
insert_values = ("value1", "value2")
execute_insert(conn, insert_query, insert_values)
# 关闭数据库连接
conn.close()
在上面的示例中,我们首先定义了一个connect_to_sql_server函数,它使用pyodbc.connect方法连接到SQL Server数据库。您需要提供正确的服务器名称、数据库名称、用户名和密码。
然后,我们定义了一个execute_query函数,它接受一个连接对象和查询语句作为参数,并使用cursor.execute方法执行查询。我们使用cursor.fetchall方法获取查询结果,并在示例中打印每一行。
接下来,我们定义了一个execute_insert函数,它接受一个连接对象、插入语句和值作为参数,并使用cursor.execute方法执行插入操作。我们使用conn.commit方法提交事务,并在示例中插入了一行数据。
最后,我们连接到SQL Server数据库,执行查询和插入操作,并关闭数据库连接。
请确保将your_server_name、your_database、your_username、your_password、your_table等替换为实际的数据库凭据和表名。确保您已经安装了pyodbc库,并且已经安装了适当的SQL Server驱动程序。
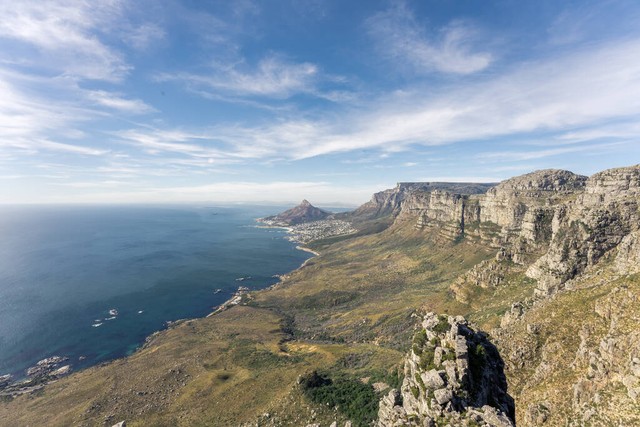
猜你喜欢
- 2024-12-16 sqlserver 测试
- 2024-12-16 Python如何解析SQL语句并转换为Python对象,SQLParse类库的使用
- 2024-12-16 Star 4.7k!纯Python开发!自称目前最快的纯Python SQL解析器!
- 2024-12-16 Python 学习 第17篇:sqlalchemy读写SQL
- 2024-12-16 盘点一个通过python大批量插入数据到数据库的方法
- 2024-12-16 python如何操作SQL Server数据库?
- 2024-12-16 Mysql四:常用查询语句(十种),一般情况够用了
- 2024-12-16 在Python中使用PostgreSQL数据库
- 2024-12-16 Python操作Sqlserver数据库(单库异步执行:增删改查)
- 07-01Python列表创建操作与遍历指南(用python创建一个列表)
- 07-01Python语言学习实战-内置函数filter()的使用(附源码)
- 07-01Python基础编程20例之七:filter过滤,筛选奇数
- 07-01使用Python过滤指定进程的技巧(python中怎么删除列表中指定的值)
- 07-01Python列表推导式如何过滤偶数?(python过滤列表中的所有负数)
- 07-01豆瓣评分8.6 《零基础入门学习Python》零基础小白必备 建议收藏
- 07-01送PDF书 | 豆瓣9.2分,超250万Python新手的选择!蟒蛇书入门到实践
- 07-01Python 从底层结构Beautiful Soup 4(内置豆瓣电影排行爬取案例)
- 272℃Python短文,Python中的嵌套条件语句(六)
- 271℃python笔记:for循环嵌套。end=""的作用,图形打印
- 270℃PythonNet:实现Python与.Net代码相互调用!
- 264℃Python操作Sqlserver数据库(多库同时异步执行:增删改查)
- 264℃Python实现字符串小写转大写并写入文件
- 123℃原来2025是完美的平方年,一起探索六种平方的算吧
- 105℃Python 和 JavaScript 终于联姻了!PythonMonkey 要火?
- 101℃Ollama v0.4.5-v0.4.7 更新集合:Ollama Python 库改进、新模型支持
- 最近发表
-
- Python列表创建操作与遍历指南(用python创建一个列表)
- Python语言学习实战-内置函数filter()的使用(附源码)
- Python基础编程20例之七:filter过滤,筛选奇数
- 使用Python过滤指定进程的技巧(python中怎么删除列表中指定的值)
- Python列表推导式如何过滤偶数?(python过滤列表中的所有负数)
- 豆瓣评分8.6 《零基础入门学习Python》零基础小白必备 建议收藏
- 送PDF书 | 豆瓣9.2分,超250万Python新手的选择!蟒蛇书入门到实践
- Python 从底层结构Beautiful Soup 4(内置豆瓣电影排行爬取案例)
- python实现爬取豆瓣电影Top250(python爬取豆瓣电影的流程)
- 基于Python+Flask+SQLite的豆瓣电影可视化系统
- 标签列表
-
- python中类 (31)
- python 迭代 (34)
- python 小写 (35)
- python怎么输出 (33)
- python 日志 (35)
- python语音 (31)
- python 工程师 (34)
- python3 安装 (31)
- python音乐 (31)
- 安卓 python (32)
- python 小游戏 (32)
- python 安卓 (31)
- python聚类 (34)
- python向量 (31)
- python大全 (31)
- python次方 (33)
- python桌面 (32)
- python总结 (34)
- python浏览器 (32)
- python 请求 (32)
- python 前端 (32)
- python验证码 (33)
- python 题目 (32)
- python 文件写 (33)
- python中的用法 (32)