网站首页 > 技术文章 正文
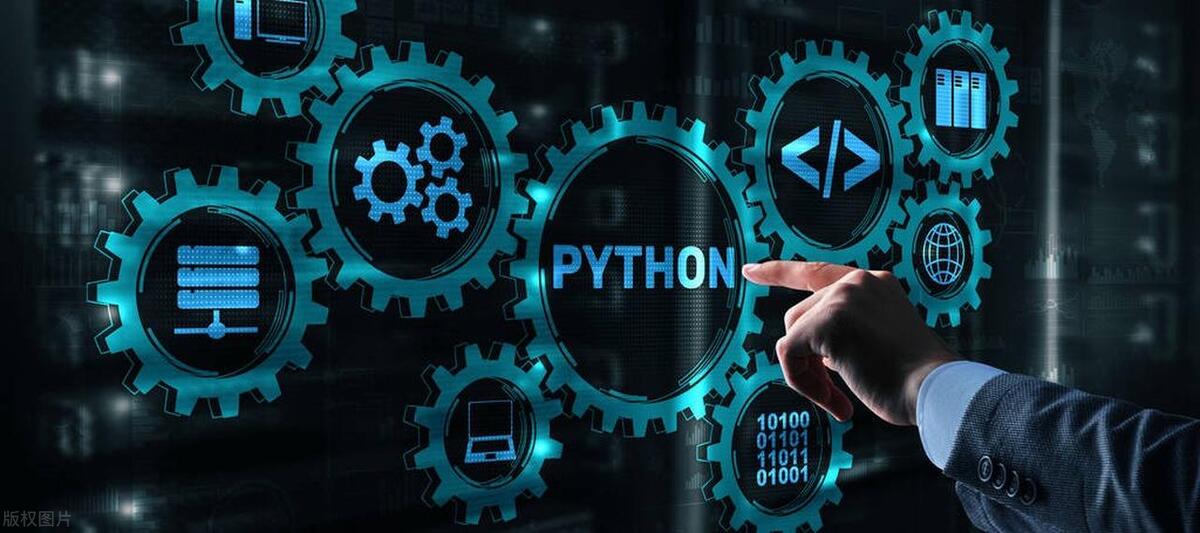
缓存数据:
将数据存储在Redis中,以避免频繁地访问数据库。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 设置缓存数据
r.set('key', 'value')
# 获取缓存数据
value = r.get('key')
print(value)
计数器:
使用Redis的原子操作实现计数器功能。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 增加计数器
r.incr('counter')
# 获取计数器值
counter = r.get('counter')
print(counter)
消息队列:
使用Redis的列表数据结构实现简单的消息队列。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 将消息加入队列
r.lpush('queue', 'message1')
r.lpush('queue', 'message2')
r.lpush('queue', 'message3')
# 获取队列中的消息
message = r.rpop('queue')
print(message)
发布订阅:
使用Redis的发布订阅功能实现消息的广播和订阅。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 发布消息
r.publish('channel', 'message')
# 订阅消息
pubsub = r.pubsub()
pubsub.subscribe('channel')
for message in pubsub.listen():
print(message)
分布式锁:
使用Redis的SETNX命令实现分布式锁。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 获取锁
lock_acquired = r.setnx('lock', '1')
if lock_acquired:
# 执行临界区代码
print('Lock acquired')
# 释放锁
r.delete('lock')
限制请求频率:
使用Redis的有序集合和过期时间实现请求限制。
import redis
import time
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 设置请求限制
r.zadd('requests', {time.time(): 'request1'})
r.zadd('requests', {time.time(): 'request2'})
# 获取最近一分钟的请求数量
current_time = time.time()
one_minute_ago = current_time - 60
requests_count = r.zcount('requests', one_minute_ago, current_time)
print(requests_count)
# 清理过期的请求记录
r.zremrangebyscore('requests', 0, one_minute_ago)
排行榜:
使用Redis的有序集合实现排行榜功能。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 设置用户积分
r.zadd('leaderboard', {'user1': 100, 'user2': 200, 'user3': 300})
# 获取排行榜前三名用户
leaderboard = r.zrevrange('leaderboard', 0, 2, withscores=True)
print(leaderboard)
地理位置查询:
使用Redis的地理位置数据结构实现位置查询功能。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 设置地理位置
r.geoadd('locations', 13.361389, 38.115556, 'Palermo')
r.geoadd('locations', 15.087269, 37.502669, 'Catania')
# 查询附近的位置
nearby_locations = r.georadius('locations', 15, 37, 200, unit='km')
print(nearby_locations)
分布式缓存:
使用Redis的集群功能实现分布式缓存。
from rediscluster import RedisCluster
# 连接到Redis集群
startup_nodes = [{'host': 'localhost', 'port': '7000'}, {'host': 'localhost', 'port': '7001'}]
r = RedisCluster(startup_nodes=startup_nodes, decode_responses=True)
# 设置缓存数据
r.set('key', 'value')
# 获取缓存数据
value = r.get('key')
print(value)
会话管理:
使用Redis的哈希数据结构存储会话数据。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 设置会话数据
r.hset('session1', 'username', 'user1')
r.hset('session1', 'email', 'user1@example.com')
# 获取会话数据
username = r.hget('session1', 'username')
email = r.hget('session1', 'email')
print(username, email)
分布式计算:
使用Redis的Lua脚本功能实现分布式计算任务。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 定义Lua脚本
lua_script = """
local result = {}
for i = 1, ARGV[1] do
result[i] = tonumber(ARGV[i + 1]) * tonumber(ARGV[i + ARGV[1] + 1])
end
return result
"""
# 执行Lua脚本
result = r.eval(lua_script, 0, 2, 10, 20)
print(result)
数据持久化:
使用Redis的RDB和AOF持久化功能将数据保存到磁盘。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 开启RDB持久化
r.config_set('save', '3600 1')
# 开启AOF持久化
r.config_set('appendonly', 'yes')
分布式锁的自动续期:
使用Redis的SET命令结合Lua脚本实现分布式锁的自动续期。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 获取锁
lock_acquired = r.set('lock', '1', ex=10, nx=True)
if lock_acquired:
# 启动自动续期
lua_script = """
if redis.call('get', KEYS[1]) == ARGV[1] then
return redis.call('expire', KEYS[1], ARGV[2])
else
return 0
end
"""
r.eval(lua_script, 1, 'lock', '1', 10)
列表操作:
使用Redis的列表数据结构实现列表操作(如推入元素、弹出元素)。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 推入元素
r.lpush('list', 'element1')
r.lpush('list', 'element2')
# 弹出元素
element = r.rpop('list')
print(element)
单点登录:
使用Redis的字符串数据结构和过期时间实现单点登录功能。
import redis
# 连接到Redis服务器
r = redis.Redis(host='localhost', port=6379, db=0)
# 设置登录状态
r.setex('session1', 3600, 'user1')
# 获取登录状态
session = r.get('session1')
print(session)
- 上一篇: CASS11免狗不挑电脑绑定
- 下一篇: 实在智能RPA:Python开发RPA如何让繁琐工作自动化
猜你喜欢
- 2024-12-17 Python 中双冒号“::”是什么运算符,有什么功能
- 2024-12-17 一文了解 Python 中的新功能:match-case 多分支选择语句
- 2024-12-17 Python中实现线程和多线程开发以及线程安全功能示例
- 2024-12-17 Python3.6-3.10发布时间及主要新增功能
- 2024-12-17 用Python实现Wake On Lan远程开机功能
- 2024-12-17 python pillow图像处理功能及应用
- 2024-12-17 Python range() 函数的功能增强版 arange()、linspace()
- 2024-12-17 python每天学习一点点(模拟10086查询功能简易版)
- 2024-12-17 Python 3.13 中5 个新增的功能将改变您的编码方式
- 2024-12-17 用python实现图像查找功能
- 05-25Python 3.14 t-string 要来了,它与 f-string 有何不同?
- 05-25Python基础元素语法总结
- 05-25Python中的变量是什么东西?
- 05-25新手常见的python报错及解决方案
- 05-2511-Python变量
- 05-2510个每个人都是需要知道Python问题
- 05-25Python编程:轻松掌握函数定义、类型及其参数传递方式
- 05-25Python基础语法
- 257℃Python短文,Python中的嵌套条件语句(六)
- 257℃python笔记:for循环嵌套。end=""的作用,图形打印
- 256℃PythonNet:实现Python与.Net代码相互调用!
- 251℃Python操作Sqlserver数据库(多库同时异步执行:增删改查)
- 251℃Python实现字符串小写转大写并写入文件
- 106℃原来2025是完美的平方年,一起探索六种平方的算吧
- 91℃Python 和 JavaScript 终于联姻了!PythonMonkey 要火?
- 81℃Ollama v0.4.5-v0.4.7 更新集合:Ollama Python 库改进、新模型支持
- 最近发表
- 标签列表
-
- python中类 (31)
- python 迭代 (34)
- python 小写 (35)
- python怎么输出 (33)
- python 日志 (35)
- python语音 (31)
- python 工程师 (34)
- python3 安装 (31)
- python音乐 (31)
- 安卓 python (32)
- python 小游戏 (32)
- python 安卓 (31)
- python聚类 (34)
- python向量 (31)
- python大全 (31)
- python次方 (33)
- python桌面 (32)
- python总结 (34)
- python浏览器 (32)
- python 请求 (32)
- python 前端 (32)
- python验证码 (33)
- python 题目 (32)
- python 文件写 (33)
- python中的用法 (32)