网站首页 > 技术文章 正文
什么是 Python 实例?
Python 中,实例是类的特定实例,表示具有唯一数据和行为的对象。类用作创建实例、定义其属性和方法的蓝图。实例允许我们基于单个类创建多个对象,每个对象都有自己的一组数据和功能。
在 Python 中创建实例
要在 Python 中创建实例,首先需要定义一个类。该类定义要创建的对象的结构和行为。定义类后,可以实例化它以创建单个实例。
考虑一个简单类的示例并创建它的实例:Person
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
# Creating instances of the Person class
person1 = Person("Alice", 30)
person2 = Person("Bob", 25)
在此示例中,创建了该类的两个实例,分别表示两个人 Alice 和 Bob。Person
实例属性和方法
Python 中的每个实例都可以有自己的一组属性和方法。属性是存储特定于实例的数据的变量,而方法是定义实例行为的函数。为了访问属性和方法,使用点表示法。
class Car:
def __init__(self, make, model):
self.make = make
self.model = 模型
def start_engine(self):
return f"The {self.make} {self.model}'s engine is now running."# Creating an instance of the Car class
my_car = Car("Toyota", "Corolla")# Accessing instance attributes
print(my_car.make) # Output: Toyota# Calling instance methods
print(my_car.start_engine()) # Output: The Toyota Corolla's engine is now running.
使用构造函数初始化实例
在 Python 中,方法__init__用作初始化实例的构造函数。创建实例时会自动调用它。构造函数允许设置实例属性的初始值。
class Circle:
def __init__(self, radius):
self.radius = radius self.area = 3.14 * radius * radius
# Creating an instance of the Circle class
circle1 = Circle(5)# Accessing instance attributes
print(circle1.radius) # Output: 5# Calculating the area using instance attributes
print(circle1.area) # Output: 78.5
Python 实例中的 Self
self关键字引用类中的实例本身。用于访问和修改实例属性,调用实例方法。在类中定义方法时,必须将self参数作为第一个参数包含在内。
class Student:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
return f"Hi, I am {self.name}, and I am {self.age} years old."# Creating an instance of the Student class
student1 = Student("John", 20)# Calling the introduce method
print(student1.introduce()) # Output: Hi, I am John, and I am 20 years old.
Python 中的访问修饰符
在 Python 中,访问修饰符用于控制类中属性和方法的可见性。三个主要的访问修饰符是 public、protected 和 private。
- 公共属性/方法:可从任何地方访问。
- 受保护的属性/方法:可在类及其子类中访问。
- 私有属性/方法:只能在类本身内访问。
class BankAccount:
def __init__(self, account_number, balance):
self.account_number = account_number
self._balance = balance # 受保护的属性
def _deduct_balance(self, amount): # Protected method
self._balance -= amount def show_balance(self): # Public method
return f"Account Number: {self.account_number}, Balance: ${self._balance}"# Creating an instance of the BankAccount class
account1 = BankAccount("12345", 1000)# Accessing public and protected attributes/methods
print(account1.show_balance()) # Output: Account Number: 12345, Balance: $1000
account1._deduct_balance(200) # Deducting $200 from the balance
print(account1.show_balance()) # Output: Account Number: 12345, Balance: $800
继承和 Python 实例
继承是面向对象编程中的一个基本概念。它允许一个类(子类)从另一个类(超类)继承属性和方法。子类的实例可以访问继承的属性/方法和特定的属性/方法。
class Animal:
def __init__(self, species):
self.species = species
def make_sound(self):
return "Some generic animal sound."class Dog(Animal):
def __init__(self, breed):
super().__init__("Dog")
self.breed = breed def make_sound(self):
return "Woof!"# Creating instances of the Dog class
dog1 = Dog("Labrador")
dog2 = Dog("Poodle")# Accessing attributes/methods from both classes
print(dog1.species) # Output: Dog
print(dog1.make_sound()) # Output: Woof!
print(dog2.species) # Output: Dog
print(dog2.make_sound()) # Output: Woof!
多态性和 Python 实例
多态性允许将不同类的对象视为公共超类的实例。它实现了代码的灵活性和模块化,因为不同的对象可以共享通用接口。
class Shape:
def calculate_area(self):
pass
class Square(Shape):
def __init__(self, side):
self.side = side def calculate_area(self):
return self.side * self.sideclass Circle(Shape):
def __init__(self, radius):
self.radius = radius def calculate_area(self):
return 3.14 * self.radius * self.radius# Creating instances of the Square and Circle classes
square1 = Square(5)
circle1 = Circle(3)# Calculating areas using polymorphism
print(square1.calculate_area()) # Output: 25
print(circle1.calculate_area()) # Output: 28.26
封装和 Python 实例
封装是在类中将数据和方法捆绑在一起的概念,以防止来自类外部的直接访问。它确保对象的内部状态是安全的,不会被无意中修改。
class BankAccount:
def __init__(self, account_number, balance):
self._account_number = account_number
self._balance = balance
def deposit(self, amount):
self._balance += amount def withdraw(self, amount):
if self._balance >= amount:
self._balance -= amount
return True
else:
return False def get_balance(self):
return self._balance# Creating an instance of the BankAccount class
account1 = BankAccount("12345", 1000)# Using methods to access and modify attributes
account1.deposit(500)
print(account1.get_balance()) # Output: 1500
account1.withdraw(2000)
print(account1.get_balance()) # Output: 1500 (Insufficient balance, withdrawal failed)
Python 中的方法重写
方法重写允许子类为已在其超类中定义的方法提供特定的实现。当实例属于子类类型时,将调用子类中重写的方法,而不是超类中的方法。
class Animal:
def make_sound(self):
return “Some generic animal sound.”
class Cat(Animal):
def make_sound(self):
return "Meow!"# Creating instances of the Animal and Cat classes
animal1 = Animal()
cat1 = Cat()# Using method overriding
print(animal1.make_sound()) # Output: Some generic animal sound.
print(cat1.make_sound()) # Output: Meow!
类变量与实例变量
在 Python 中,类变量在类的所有实例之间共享,而实例变量特定于每个实例。类变量在方法外部定义,使用类名进行访问,而实例变量在方法中定义,并使用实例进行访问。
class Circle:
# 类变量
pi = 3.14
def __init__(self, radius):
# Instance variable
self.radius = radius def calculate_area(self):
return Circle.pi * self.radius * self.radius# Creating instances of the Circle class
circle1 = Circle(5)
circle2 = Circle(3)# Accessing class and instance variables
print(circle1.calculate_area()) # Output: 78.5
print(circle2.calculate_area()) # Output: 28.26
实例方法与类方法
实例方法使用参数self定义并对实例本身进行操作,而类方法cls使用参数定义并对整个类进行操作。类方法由装饰器@classmethod表示。
class MyClass:
class_variable = 10
def __init__(self, instance_variable):
self.instance_variable = instance_variable def instance_method(self):
return f"Instance variable value: {self.instance_variable}" @classmethod
def class_method(cls):
return f"Class variable value: {cls.class_variable}"# Creating an instance of the MyClass class
my_instance = MyClass(20)# Using instance and class methods
print(my_instance.instance_method()) # Output: Instance variable value: 20
print(MyClass.class_method()) # Output: Class variable value: 10
Python 中的单例设计模式
单一实例设计模式确保类只有一个实例,并提供对该实例的全局访问点。在想要限制类的实例数的情况下,它可能很有用。
class Singleton:
_instance = 无
def __new__(cls):
if cls._instance is None:
cls._instance = super().__new__(cls)
return cls._instance# Creating instances of the Singleton class
singleton1 = Singleton()
singleton2 = Singleton()# Both instances are the same
print(singleton1 == singleton2) # Output: True
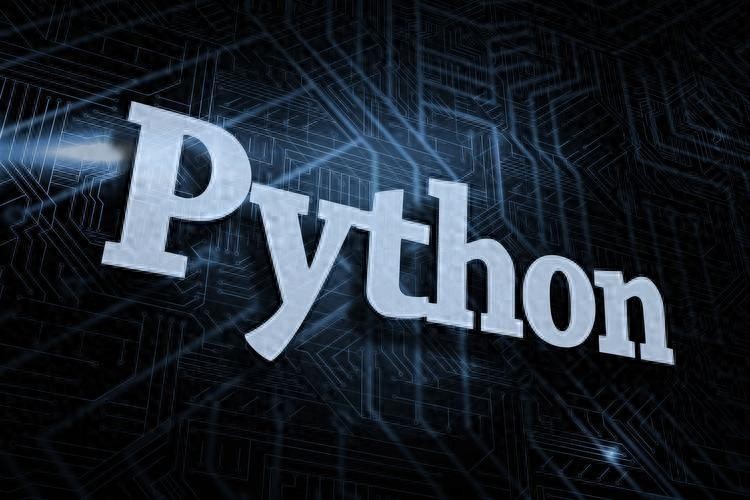
猜你喜欢
- 2024-12-12 分享3个干货满满的Python实战项目,点赞收藏
- 2024-12-12 python爬虫经典案例,看完这一篇就够了
- 2024-12-12 收藏!20条非常实用的Python代码实例
- 2024-12-12 Python一行代码能做什么,30个实用案例代码详解
- 05-25Python 3.14 t-string 要来了,它与 f-string 有何不同?
- 05-25Python基础元素语法总结
- 05-25Python中的变量是什么东西?
- 05-25新手常见的python报错及解决方案
- 05-2511-Python变量
- 05-2510个每个人都是需要知道Python问题
- 05-25Python编程:轻松掌握函数定义、类型及其参数传递方式
- 05-25Python基础语法
- 257℃Python短文,Python中的嵌套条件语句(六)
- 257℃python笔记:for循环嵌套。end=""的作用,图形打印
- 256℃PythonNet:实现Python与.Net代码相互调用!
- 251℃Python操作Sqlserver数据库(多库同时异步执行:增删改查)
- 251℃Python实现字符串小写转大写并写入文件
- 106℃原来2025是完美的平方年,一起探索六种平方的算吧
- 91℃Python 和 JavaScript 终于联姻了!PythonMonkey 要火?
- 81℃Ollama v0.4.5-v0.4.7 更新集合:Ollama Python 库改进、新模型支持
- 最近发表
- 标签列表
-
- python中类 (31)
- python 迭代 (34)
- python 小写 (35)
- python怎么输出 (33)
- python 日志 (35)
- python语音 (31)
- python 工程师 (34)
- python3 安装 (31)
- python音乐 (31)
- 安卓 python (32)
- python 小游戏 (32)
- python 安卓 (31)
- python聚类 (34)
- python向量 (31)
- python大全 (31)
- python次方 (33)
- python桌面 (32)
- python总结 (34)
- python浏览器 (32)
- python 请求 (32)
- python 前端 (32)
- python验证码 (33)
- python 题目 (32)
- python 文件写 (33)
- python中的用法 (32)